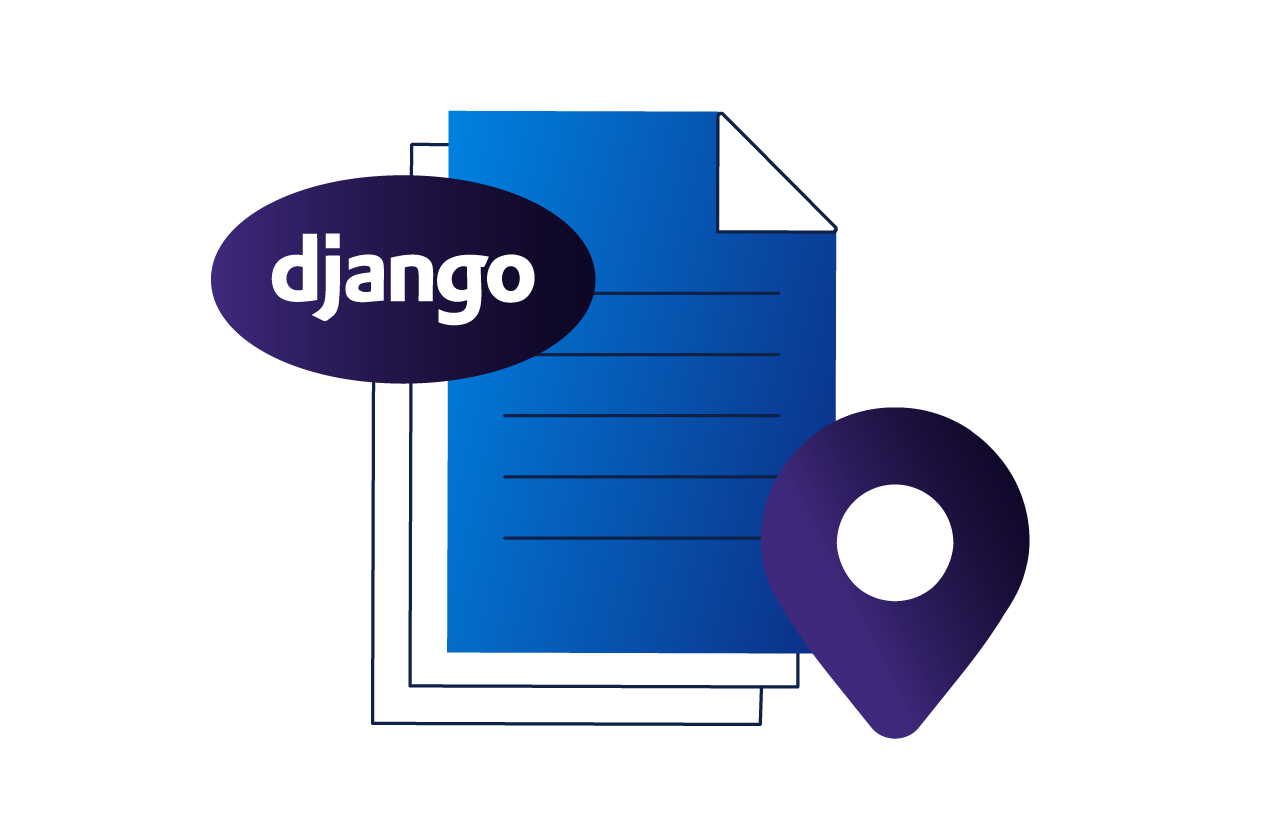
The Conclusive Guide to Django Localization
- What is Localization?
- Django Localization
- Using files VS OTA updates
- Django Localization Using Transifex Νative (Fileless Approach)
- Setting up the Django SDK
- Pushing Content to Transifex
- Pushing Content to the Django App
- Language Picker
- Django Localization in Action
- Why Go With Fileless/Native Over Using Localization Files?
Due to its popularity, Django is often used to develop applications serving audiences spanning different localities and regions, which is where Django localization comes in.
In order to guarantee the best user experience for all your users, it is best that you tailor your application to match your users’ language and cultural preferences.
In this article, we will briefly highlight forms of localization and how you can install and configure the Django SDK in your Django projects, as well as how you can leverage it to perform localization in your projects.
What is Localization?
Essentially, localization is the process of taking a product, service, or content in its original form and adapting it to fit the standards, cultural, linguistic, or laws and regulations of people in a given locale.
Typically, localization involves translating a product or service to a local dialect that people in a specific market understand. However, it is much more than that. Localization goes beyond just text translation and can involve other forms of media, such as images and videos.
Localization is important for a number of reasons. First and foremost, it allows companies to expand their reach and tap into new markets. By making their products and services available in different languages and cultures, they can appeal to a wider audience and potentially increase their sales.
Django Localization
Django has built-in support for translating text and formatting dates, numbers, times, and time zones. This is made possible because Django does the following:
- Django allows developers and template authors to specify parts of their applications. Parts that they want to translate and format for local languages and cultures.
- Django supports hooks, also called translation strings, which tell Django which text should be translated. So then you can easily localize your web applications.
While Django provides a rich set of i18 tools, it has several limitations, such as locale restrictions. However, with Transifex, you can streamline your Django localization and translation efforts by leveraging its advanced features and integrations.
Using files VS OTA updates
There are different approaches to localizing your Django project using Transifex. Depending on your project needs and size, you can choose to use:
- File-based localization – Extract the translatable strings from your source code into translation files, which are then uploaded to a translation management platform such as Transifex. These translation files can be in various formats, such as PO (Portable Object) or XLIFF (XML Localization Interchange File Format).
- Fileless localization – Store the translatable strings directly in the translation management platform, all without creating any translation files. Instead, use the platform’s API to access and update the strings OTA by using a CDN.
In this article, we will focus on fileless localization using the Django Transifex native SDK.
Django Localization Using Transifex Νative (Fileless Approach)
Transifex Native is a collection of tools that allow for easy localization of your applications by installing framework-specific SDKs.
For instance, you need to install the Django SDK when working with Django projects. Once you have installed an SDK, you can push or pull content for localization directly with Transifex without the need for a file.
While Django provides internationalization hooks that are on by default, Transifex Native also offers its own translation hook commands for Django templates, {% t %}, and Python views, t().
The Transifex Native localization framework utilizes the ICU syntax to specify localization rules. It also allows you to specify metadata such as context, tags, and character limits for individual strings.
The Transifex Django SDK is a comprehensive toolkit designed to seamlessly integrate with the Django framework.
It includes features for mapping source strings for translation, retrieving translated strings, and displaying translations in templates or views. Additionally, the SDK offers Command Line Interface (CLI) commands to help push translations to Transifex and migrate existing template code to the new syntax.
Setting up the Django SDK
Prerequisites.
- Python 2.7, 3.5+
- Django 1.11+
To use the Transifex Django SDK, you will need to sign up for a Transifex account or login into an existing one. Once logged in, you’ll need to create a new Transifex native project from within the Transifex application.
To install the Django SDK run the command below on the terminal.
pip install transifex-python
To be able to use the Transifex Native SDK with the Transifex platform through the Transifex Content Delivery Service, you require requires certain credentials to do so.
These credentials include a project_secret, which is related to your Transifex project and is used for pushing source content to Transifex, and a project_token, which is also related to your Transifex project and is used for pulling translations from Transifex.
To obtain these credentials, we first need to create a new native project and choose the source and target languages.
Once you have created your project, select your SDK as Django and generate the Transifex native credentials that will allow the Django SDK to communicate with Transifex.
Copy the transifex native token and secret since we will need them in the subsequent steps for configuration.
Navigate to the settings file of your Django projects and add the entries below to the file.
INSTALLED_APPS = [
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
'accounts',
'django_filters',
'transifex.native.django',
]
LANGUAGE_CODE = 'en' # replace with your project's source language
USE_I18N = True
USE_L10N = True
# Replace with the proper values for the Transifex project token and secret,
# as found in the Transifex UI under your project
TRANSIFEX_TOKEN = '1/babe7cc10a86f499fdfe70826afe20fc84e2c124' # used for pulling translations from Transifex
TRANSIFEX_SECRET = '1/d93c5646b2f97642adb33fbb9fec9dc93aa9927e' # used for pushing source content to Transifex
TRANSIFEX_SYNC_INTERVAL = 5 # used for defining the daemon running interval in seconds
Once we have configured the Django SDK, you can now start pushing, pulling, and displaying the translations to your Django application.
Pushing Content to Transifex
Now that you’ve configured your SDK, we can directly push our content to Transifex without needing external files. You can use it inside Django templates and views.
For instance, to use the Transifex SDK in our templates, we need to add the following template tags on the top of your HTML files.
{% load i18n %}
{% load transifex %}
Now make strings and variables in the HTML code translatable by using any of the two template tags below.
{% t %}
{% ut %}
For instance, here is a section of a demo application wrapped using one of the tags above.
<div class="col-md-7">
<h5>{% t "LAST 5 ORDERS" %}</h5>
<hr>
<div class="card card-body">
<hr>
<table class="table table-sm">
<tr>
<th>{% t "Product" %}</th>
<th>{% t "Date Ordered" %}</th>
<th>{% t "Status" %}</th>
<th>{% t "Update" %}</th>
<th>{% t "Remove" %}</th>
{% for order in orders %}
<tr>
<td>{% t "Dishes" %} </td>
<td>{{order.date_created}}</td>
<td>{% t "Delivered" %}</td>
<td><a class = "btn btn-sm btn-info" href="">{% t "Update" %} </a> </td>
<td><a class = "btn btn-sm btn-danger" href="">{% t "Delete" %}</a></td>
</tr>
{% endfor %}
We can also mark translatable strings inside out Django views by importing a function at the top of our python file and then before using it to wrap strings. Here is a snippet of how that can be done.
from transifex.native.django import t
from django.shortcuts import render, redirect
from django.forms import inlineformset_factory
from .filters import OrderFilter
from django.contrib import messages
from django.contrib.auth import authenticate, login, logout
from django.contrib.auth.decorators import login_required
def name_of_the_view(request):
output = t("Welcome aboard!")
return render(output)
Now that you have marked all your strings inside your templates and views, it is time to push the to Transifex for translation. Use the command below on your terminal.
python3 manage.py transifex push
The command above scans all your project files and collects all translatable strings before pushing them to Transifex.
##############################################################
Transifex Native: Parsing files to detect translatable content
Processed 35 files and found 31 translatable strings in 2 of them.
Pushing 24 unique translatable strings to Transifex...
Processing........
Successfully pushed strings to Transifex.
Created strings: 4
Skipped strings: 20
Now that we have successfully pushed our Translatable content to Transifex, it is time to translate that content before we can pull it back to our site.
Here is what our Django app looks like before localization.
Now, if you navigate to your Transifex project and refresh, you should see the new strings uploaded to your project, as shown below.
And once you are at this stage, you can translate your content by relying either on translators or machine translation. Ideally, a combination of both.
Pushing Content to the Django App
Transifex Native allows you to continuously retrieve translations for your application “over the air” (OTA). This means that you do not need to restart your server. Nor do you have to deploy new code in order to access the latest translations.
When the application is first launched, the integration will populate its internal memory with the current state of translations from Transifex. A background thread is also run to periodically re-fetch translations and update the internal memory accordingly.
Language Picker
To select a different language, we will implement a language picker that you should see on the page we are localizing. This is a simple HTML form that you can add to any HTML file and does not need CSS or JS to work.
{% load i18n %}
{% load transifex %}
<form action="{% url 'set_language' %}" method="post">{% csrf_token %}
<input name="next" type="hidden" value="/" />
<select name="language">
{% get_current_language as LANGUAGE_CODE %}
{% get_available_languages as LANGUAGES %}
{% get_language_info_list for LANGUAGES as languages %}
{% for language in languages %}
<option value="{{ language.code }}"{% if language.code == LANGUAGE_CODE %} selected{% endif %}>
{{ language.name_local }} ({{ language.code }})
</option>
{% endfor %}
</select>
<input type="submit" value="Go" />
</form>
For the language hook above to work, we are going to modify our project routes as shown below.
from django.contrib import admin
from django.urls import path, include
urlpatterns = [
path('admin/', admin.site.urls),
path('', include('accounts.urls')),
path(r'^i18n/', include('django.conf.urls.i18n')), #remember to add this here
]
Now start up the Django application with the command below.
python3 manage.py runserver
Django Localization in Action
By default, Transifex Native automatically displays content in the language currently selected in your Django application. In this case, our default language is English(en).
Now, if we select Arabic (ar) our Django application should automatically be translated to Arabic, as shown below.
Since I also selected French as another target language, I can also simply change our Django app to French using the language picker we created.
However, note that you need to have also translated your strings on Transifex as we did earlier. This process does not require us to restart or modify the Django configurations we made earlier.
In conclusion, the Transifex Native Django SDK is a powerful tool that allows developers to easily integrate Transifex into their Django projects.
With the Transifex Native Django SDK, developers can map source strings for translation, retrieve translated strings, and display translations in templates or views.
The SDK also provides command line interface (CLI) commands for pushing translations to Transifex. You also have built-in functions to help developers migrate existing Django apps from Django i18n to Transifex Native.
To use the Transifex Native Django SDK, you need to have a Transifex account and a Django project. Then you’ll also have to configure your Django project to use the SDK.
Overall, the Transifex Native Django SDK is a valuable resource for developers looking to manage the localization of their Django projects with Transifex.
Why Go With Fileless/Native Over Using Localization Files?
This method allows you to send individual batches of phrases for localization, which you can add or update in a pool of phrases accessible through the Content Delivery Service.
By treating these phrases as individual units of content, you can combine content from various applications and access it as a single pool of phrases. Utilizing this fileless transmission, your applications can request batches of translated content for a localized version.
File-based localization has been in use for a long time. But you may find it problematic when working on large projects involving large teams. Fast feature releases and deployments are key in today’s complex development environment.
With the Fileless localization approach, you can pull and push content between development and prod environments. All without requiring complete redeployments.
Other key benefits of the fileless localization using Transifex native over the file-based approach include the following:
- Localization becomes part of the development cycle; thus, there are no delays or additional steps to manage localization.
- Framework-specific SDKs allow you to manage content by following a unified syntax and formatting, which is the ICU syntax.
- Ability to push content directly from developer workstations so that it is immediately available for localization and pull it right back from the Transifex application using it.
- Unified content management allows developers to push content to production by working on parallel branches.
- Multiple applications can add content in parallel to the same project, leverage the same process for the same strings, and seamlessly sync updates Over the Air OTA without having to bother with files.
Related posts
React Localization With Transifex Native: All you Need to Know
Python Localization: How to Localize your Python App
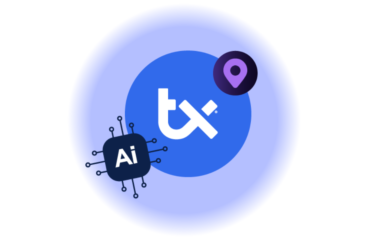
AI Localization: Everything You Need to Know
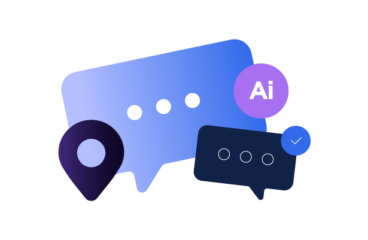